CS50 — Week 2 (Arrays):
Week 2 of CS50 develops on the foundations laid by Week 1 and introduces new features of C. The central focus of Week 2 was a data structure called arrays. An array in C allows the user to store a string (a series of chars) or a number with more than one digit as contiguous bytes of memory and allows you to access individual characters within that array. Moreover, the amount of space required to store the different types is touched on in the lecture and how that is stored in our computer’s random access memory (RAM).
Another benefit of arrays is how they simplify our code and make the entire program more efficient especially when compared to creating a variable for each and every input.
Week 2 also elaborates on command line arguments and how main can accept two variables (argument count and argument vector) rather than be void. Argument count is an integer that represents the number of words the user types at prompt. Argument vector is a fancy way of saying list and is a variable that is going to store all of the strings that a human types at the prompt in an array.
Lab 2 revolved around a game of Scrabble whereby the points for each letter were assigned using an array. The program would take a word for Player 1 and Player 2 and calculate the total score of that word and print the name of the winner or declared a draw if the scores were equal. It was intellectually challenging to come up with a solution on how to assign the correct number of points to the corresponding letter regardless of their case.
The solution to this was to subtract 65 and 97 if the letters were uppercase or lowercase respectively. Following this, it was a case of allocating it to the position in the array and then assigning it the points from the array.

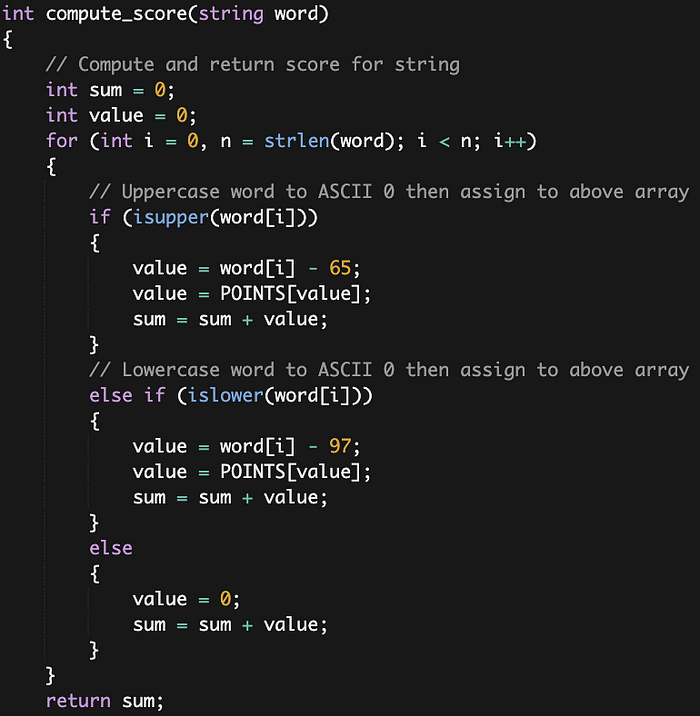
The problem sets for Week 2 were very difficult and a pattern began to emerge regarding how the CS50 course is structured. The lectures provide you with the information and base level of understanding required to interpret the problem and begin to think computationally. The onus is then on the individual to research and study further into the key topics and develop a firmer understanding of advanced examples.
The purpose of Readability was to accept an input of text from the user and to grade its reading level according to the Coleman-Liau index. This linked back to the lecture because it required iterating over the input (string) and count the number of letters, words and sentences within the input text. From there, the figures would then be inserted into the formula to then print the grade.

Caesar is a program which encrypts user input (plain text) and prints out the cipher text. It utilises both arrays and command line arguments as it iterates through the input and ciphers it through a formula and the argument vector acts as a key to know how many places in the alphabet to shift the plain text. For example, if argv were 1 and if the user were to input “hi”, the program would print “ij”.
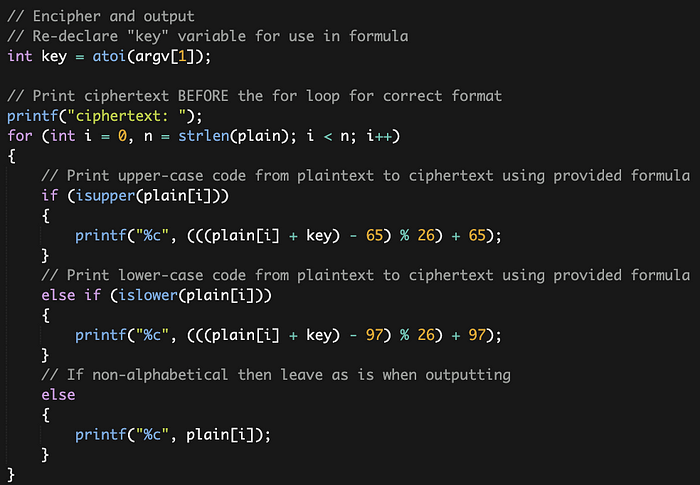
On the whole, Week 2 was enjoyable and really taught me a lot about arrays and how to iterate over the data structure. It was a long process to solve how to take the case of the letters within the string into account and handle it accordingly. However, I have developed a better grasp on the concepts of arrays through exploring their potential and using them throughout the week’s lab and problem sets.
Thank you for reading!